Without a question, the Microsoft.NET ecosystem has grown over the years and each new version introduces new features. Microsoft announced.Net 8 last month in honor of the 20th anniversary of Net.
Native AOT in ASP.NET Core
Hold onto your hats! Native AOT has finally arrived to ASP.NET Core apps! This means that when you publish an app, it will be compiled with native code. No more need for the .NET runtime on the machine!
Why native AOT rocks in ASP.NET Core
The combination of native AOT with ASP.NET Core leads to impressive results: smaller footprint, blazing-fast startup, and reduced memory usage.
These advantages are particularly noticeable in situations where multiple instances are running, such as cloud infrastructure and large-scale services. It’s truly remarkable, isn’t it?
This new feature offers some impressive advantages that will make developers’ lives easier and more efficient. Here are the benefits you can expect when using Native AOT:
- Smaller disk footprint: This means that one executable contains the program and used code from external dependencies. In this case, the deployment will increase its speed. On the other hand, the other advantage is that the container images will be much smaller compared to normal.
- Faster startup time: JThe time needed for the app to startup will be shorter thanks to not going through JIT compilation. This makes deployments with container orchestrators much smoother.
- Lower memory use: Improved scalability because the app will need to use significantly less memory than before.
In Microsoft’s tests, they tested an ASP.NET Core API app with native AOT. The results were an 87% smaller size and an 80% improved start-up time.
Minimal APIs Meet Native AOT
Get ready for a fantastic combo! Through a clever introduction of the RDG (Request Delegate Generator), Microsoft has successfully expanded the capabilities of Minimal APIs, enabling seamless integration with Native AOT support, paving the way for enhanced performance and optimization
.This means you can now enjoy a fantastic combination of these two features.
As a source generator, RDG turns those MapGet()
, MapPost()
, and other calls into RequestDelegate
s with routes during compile-time. No more runtime code generation!
Enabling native AOT publishing breathes life into RDG and enhances its performance.
You may have to do a lot of testing in case you need to calibrate for AOT or reduce the startup time of the project.. Give it a try and see the difference for yourself!
YOu can manually enable RDG in your project with <EnableRequestDelegateGenerator>true</EnableRequestDelegateGenerator>
.
Minimal APIs love JSON payloads, so the System.Text.Json
source generator steps in to handle them. Just register a JsonSerializerContext
:
// Register the JSON serializer context with DI
builder.Services.ConfigureHttpJsonOptions(options =>
{
options.SerializerOptions.AddContext<AppJsonSerializerContext>();
});
...
// Add types used in your Minimal APIs to source generated JSON serializer content
[JsonSerializable(typeof(Todo[]))]
internal partial class AppJsonSerializerContext : JsonSerializerContext
{
}
Now you’ve got a powerful mix of Minimal APIs and native AOT!
Native AOT-ready Templates
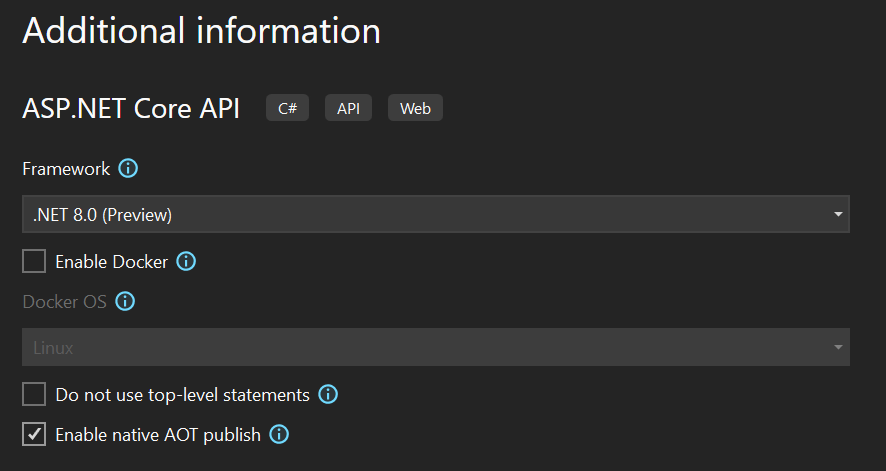
In this preview, Microsoft unveils two native AOT-enabled project templates for ASP.NET Core.
The “ASP.NET Core gRPC Service” template now has an “Enable native AOT publish” option. Tick that box, and <PublishAot>true</PublishAot>
pops up in your .csproj file.
Introducing the fresh “ASP.NET Core API” template, designed for cloud-native, API-first projects. It’s different from the “Web API” template because it:
- Only uses Minimal APIs (no MVC yet)
- Employs
WebApplication.CreateSlimBuilder
for essential features - Listens to HTTP only (cloud-native deployments handle HTTPS)
- Skips IIS Express launch profiles
- Enables JSON serializer source generator for native AOT
For example, from the dotnet CLI we have the possibility to create a new API project with native AOT:
$ dotnet new api -aot
The “ASP.NET Core API” template creates a set of instructions called Program.cs
content that help your project work better and faster from the start.
using System.Text.Json.Serialization;
using MyApiApplication;
var builder = WebApplication.CreateSlimBuilder(args);
builder.Logging.AddConsole();
builder.Services.ConfigureHttpJsonOptions(options =>
{
options.SerializerOptions.AddContext<AppJsonSerializerContext>();
});
var app = builder.Build();
var sampleTodos = TodoGenerator.GenerateTodos().ToArray();
var todosApi = app.MapGroup("/todos");
todosApi.MapGet("/", () => sampleTodos);
todosApi.MapGet("/{id}", (int id) =>
sampleTodos.FirstOrDefault(a => a.Id == id) is { } todo
? Results.Ok(todo)
: Results.NotFound());
app.Run();
[JsonSerializable(typeof(Todo[]))]
internal partial class AppJsonSerializerContext : JsonSerializerContext
{
}
Ready, set, go with native AOT templates!
Manage Request Timeouts
Now we will have a new middleware. It will mainly be in charge of managing response times in a better way.
So it will be easier to set the timeouts for different controllers or endpoints.
Let’s check the Microsoft example:
builder.Services.AddRequestTimeouts();
After that you can use UseRequestTimeouts()
:
app.UseRequestTimeouts();
For specific endpoints, use WithRequestTimeout(timeout)
When timeouts expire, HttpContext.RequestAborted
triggers, but requests won’t be forcibly stopped.
Quick Route Short Circuit
In this update, Microsoft introduces a new option for quicker route handling.
This means that any configured middleware will run before the code that handles the request, allowing for additional processing or modifications to be made before the response is sent back.
However, you might not need this for certain requests, like robots.txt or favicon.ico.
You can use the ShortCircuit()
option:
app.MapGet("/", () => "Hello World").ShortCircuit();
For quickly sending a 404 or another status code without further processing, try MapShortCircuit
:
app.MapShortCircuit(404, "robots.txt", "favicon.ico");
This efficient approach bypasses unnecessary features like authentication and CORS.
Blazor’s Handy Sections
Microsoft has added two new components, SectionOutlet
and SectionContent
, to Blazor in order to simplify the process of creating content placeholders in layouts.
With these components, developers can easily create named sections that can be filled by specific pages using unique names or object IDs.
Let’s check the Microsoft example:
@using Microsoft.AspNetCore.Components.Sections
...
<div class="top-row px-4">
<SectionOutlet SectionName="TopRowSection" />
<a href="https://docs.microsoft.com/aspnet/" target="_blank">About</a>
</div>
Also:
<SectionContent SectionName="TopRowSection">
<button class="btn btn-primary" @onclick="IncrementCount">Click me</button>
</SectionContent>
Sections make organizing content a breeze!
What is ASP.NET Hosting?
A strong web development language, ASP.Net is comparable to Java or PHP in its capabilities.
Due to its interdependence on Windows OS, ASP.net is the ideal option for the Windows platform. It is a framework for web applications that may be used to build dynamic web pages and services.
Numerous hosting firms are offering ASP.net hosting alternatives to help with this. These hosting alternatives are combined with a number of add-ons that are readily available for quick hosting and web development.
If you are looking for best ASP.NET hosting, I will recommend you to choose ASPHostPortal. ASPHostPortal has substantial infrastructure to provide good speed to your website. It also has some enhanced security features and is a good option in case budget is not a constraint.